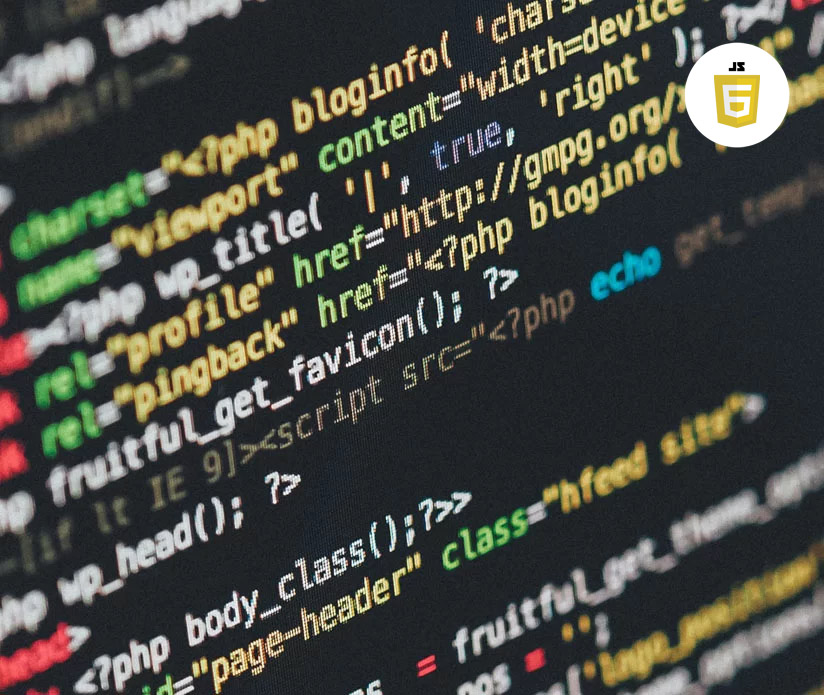
How to create a WordPress Plugin
There are several different ways a WordPress plugin can be structured but the approach that i prefer is a class based approach as it keeps all of my code organized and well structured for scalability. My approach for this example basically consists of a base file, where i define the WordPress metadata and some PHP constants, and two classes – the first of which is used to instantiate the plugin and the second class is a simple factory which registers custom post types.
Below you’ll find 3 separate code blocks which makes up the overall structure of a WordPress plugin. If you want more of an explanation on each block of code be sure to watch tutorial video – which is also posted on my Youtube channel.
Step 1. The Base file
This is the base which resides in the root folder of my plugin structure. This file is responsible for defining the WordPress metadata, several PHP constants and importing the main plugin class so we can run it.
<?php /* Plugin Name: Kindel Post Types Pack Plugin URI: https://www.kindel.ca Description: Custom Post Types for Kindel LMS Theme Version: 1.0.0 Author: Kindel Author URI: https://www.kindel.ca License: GPLv2 or later License URI: http://www.gnu.org/licenses/gpl-2.0.txt Text Domain: kindel-cpt-pack */ /* Do not access this file directly */ if ( !defined('WPINC') && !defined('ABSPATH') ) { die; } /* Constants ------------------------------------------ */ // Set the plugin version constant. if ( !defined( 'KINDEL_CPT_PACK_BASE_VERSION' ) ) { define( 'KINDEL_CPT_PACK_BASE_VERSION', '1.0.0' ); } // Set constant server path to the current plugin. (ex. /home/www/your_site/wp-content/plugins/your-plugin/) if ( !defined( 'KINDEL_CPT_PACK_BASE_PATH' ) ) { define( 'KINDEL_CPT_PACK_BASE_PATH', trailingslashit( plugin_dir_path(__FILE__) ) ); } // Set the constant URL path to the current plugin. (ex. http://example.com/wp-content/plugins/my-plugin/) if ( !defined( 'KINDEL_CPT_PACK_BASE_URI' ) ) { define( 'KINDEL_CPT_PACK_BASE_URI', trailingslashit( plugin_dir_url( __FILE__ ) ) ); } // Define the path to the plugins assets folder (if required) if ( !defined( 'KINDEL_CPT_PACK_ASSETS_URL' ) ) { define( 'KINDEL_CPT_PACK_ASSETS_URL', KINDEL_CPT_PACK_BASE_URI . 'assets'); } // Define the language domain for translation purposes if ( !defined( 'KINDEL_CPT_PACK_LANGUAGE_DOMAIN' ) ) { define( 'KINDEL_CPT_PACK_LANGUAGE_DOMAIN', 'kindel-cpt-pack'); } /* Initialize the plugin ------------------------------------------ */ if( is_admin() ){ require_once( KINDEL_CPT_PACK_BASE_PATH . 'includes/class.post-types.php' ); }
Step 2. The Main Class
The following code is the main class, which resides in a folder labelled includes, and runs all of the essential code to get the plugin working.
//Import the post type factory (used to register new post types) include_once( 'factories/class.post-type-factory.php' ); if(!class_exists("Kindel_CPT_Pack")) { class Kindel_CPT_Pack { //Define private variables if required (these are stored at the top level scope of the class) private $post_type_factory; private $courses_post_type = 'tp_courses'; private $language_domain = KINDEL_CPT_PACK_LANGUAGE_DOMAIN; private $base_path = KINDEL_CPT_PACK_BASE_PATH; public function __construct() { //Instantiate factories $this->post_type_factory = new Post_Type_Factory(); //Language support add_action('init', array($this, 'ln_load_language_support')); //Register post types add_action( 'init', array($this, 'ln_register_post_types')); //Clean up work upon deactivation add_action('deactivated_plugin', array($this, 'ln_plugin_deactivation_callback'), 10, 2); } public function ln_load_language_support() { load_plugin_textdomain( $this->language_domain, false, $this->base_path . '/languages' ); } public function ln_register_post_types() { $args = array( 'supports' => array('title', 'editor', 'excerpt', 'thumbnail'), 'rewrite' => array('slug' => 'post_courses'), ); //Register Courses CPT if ( !post_type_exists($this->courses_post_type) ) { $this->post_type_factory->register_new_post_type($this->courses_post_type, __('Course', $this->language_domain), __('Courses', $this->language_domain), $this->language_domain, $args); } } public function ln_plugin_deactivation_callback() { //Unregister post types unregister_post_type($this->courses_post_type); } } } //Instantiate the class $kindel_cpt_pack = new Kindel_CPT_Pack;
Step 3. The Post Type Factory
And the following code is the post type factory which is responsible for creating new post types. This code resides in the includes folder nested in a sub-folder labelled factories (ex. includes/factories).
if(!class_exists("Post_Type_Factory")) { class Post_Type_Factory { public function register_new_post_type($post_type, $singular_name, $plural_name, $language_domain, $args = []) { //Set up the labels for the custom post type $labels = array( 'name' => _x($plural_name, 'Post Type General Name', $language_domain), 'singular_name' => _x($singular_name, 'Post Type Singular Name', $language_domain), 'menu_name' => __($plural_name, $language_domain), 'name_admin_bar' => __($singular_name, $language_domain), 'archives' => __($singular_name . ' Archives', $language_domain), 'attributes' => __($singular_name . ' Attributes', $language_domain), 'parent_item_colon' => __('Parent ' . $singular_name . ':', $language_domain), 'all_items' => __('All ' . $plural_name, $language_domain), 'add_new_item' => __('Add New ' . $singular_name, $language_domain), 'add_new' => __('Add New', $language_domain), 'new_item' => __('New ' . $singular_name, $language_domain), 'edit_item' => __('Edit ' . $singular_name, $language_domain), 'update_item' => __('Update ' . $singular_name, $language_domain), 'view_item' => __('View ' . $singular_name, $language_domain), 'view_items' => __('View ' . $plural_name, $language_domain), 'search_items' => __('Search ' . $singular_name, $language_domain), 'not_found' => __('Not found', $language_domain), 'not_found_in_trash' => __('Not found in Trash', $language_domain), 'featured_image' => __('Featured Image', $language_domain), 'set_featured_image' => __('Set featured image', $language_domain), 'remove_featured_image' => __('Remove featured image', $language_domain), 'use_featured_image' => __('Use as featured image', $language_domain), 'insert_into_item' => __('Insert into ' . $singular_name, $language_domain), 'uploaded_to_this_item' => __('Uploaded to this ' . $singular_name, $language_domain), 'items_list' => __($plural_name . ' list', $language_domain), 'items_list_navigation' => __($plural_name . ' list navigation', $language_domain), 'filter_items_list' => __('Filter ' . $plural_name . ' list', $language_domain), ); //Merge any custom arguments with the default arguments $default_args = array( 'label' => __($singular_name, $language_domain), 'labels' => $labels, 'supports' => array('title', 'editor', 'thumbnail'), 'hierarchical' => false, 'public' => true, 'show_ui' => true, 'show_in_menu' => true, 'menu_position' => 20, 'show_in_admin_bar' => true, 'show_in_nav_menus' => true, 'can_export' => true, 'has_archive' => true, 'exclude_from_search' => false, 'publicly_queryable' => true, 'capability_type' => 'post', ); $final_args = array_merge($default_args, $args); //Register the custom post type register_post_type($post_type, $final_args); } } }
As mentioned, if you require more details on what each block of code does exactly then watch the Tutorial video posted on this page or on my Youtube channel.
If you have any questions or require help with your web project feel free to get in touch with me at leo@digitalodyssey.ca