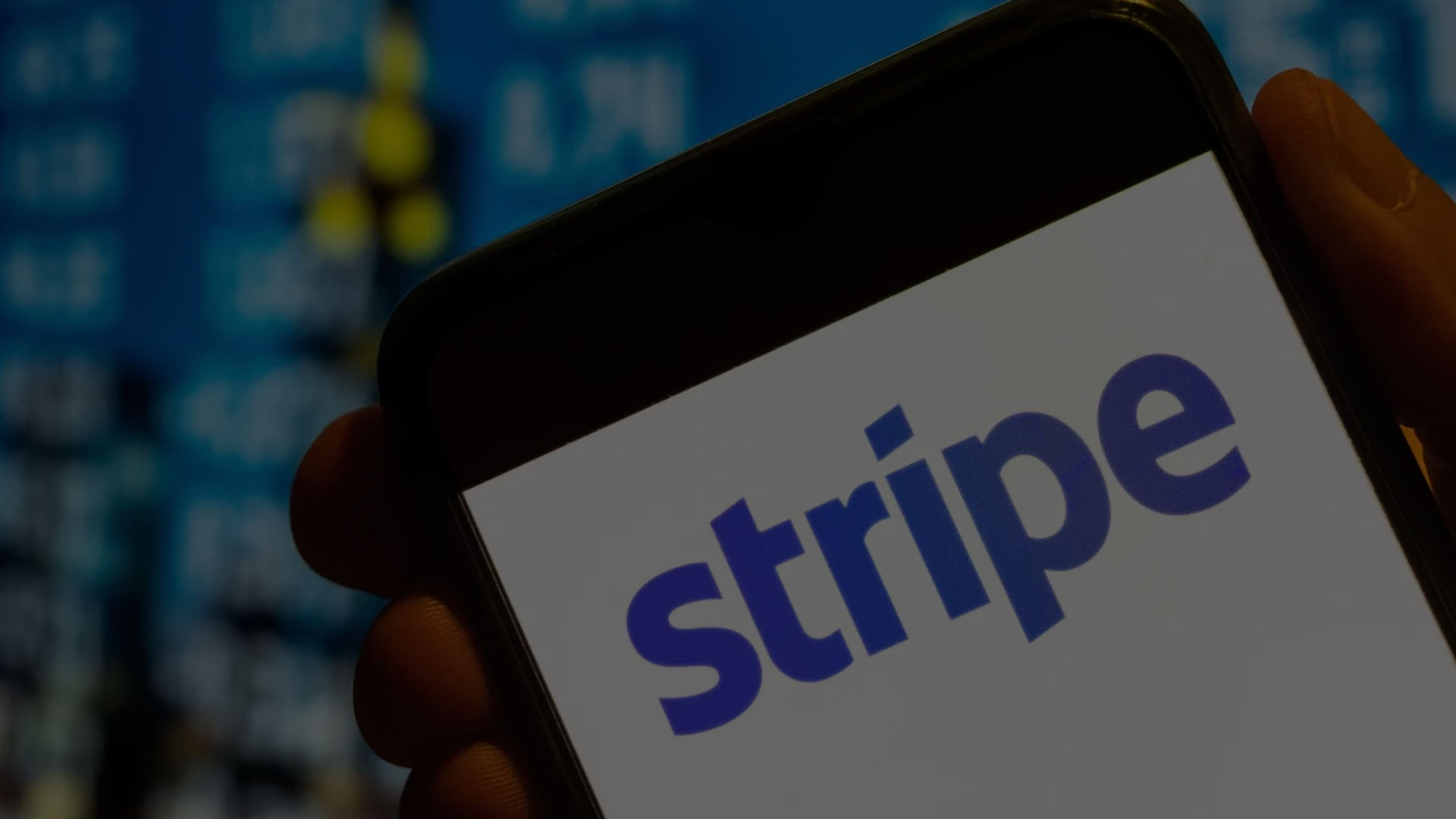
Creating a simple Stripe checkout using the Stripe API
The following PHP code is in relation to one of my recent videos which provides an overview on how to work with the Stripe API and generating a Stripe checkout session – you can watch the video posted in the sidebar.
Before trying the following code you will need to import the Stripe API library into your PHP project. Once that has been imported the following code work just fine – you will just need to change the $final_price variable and product data name value and configure both the success and cancel URL’s to your specific project requirements. The json_encode can be used to send data back to Javascript if required.
try { //STRIPE Configuration $secret_key = "sk_test_98234akdsfkjasldfjklsdfsadfdsfwnZAn31aO35nMO4Lnvx7sVVVsdlkfj234q5R1DBUvUSFe6ksTUz42345NdyQtmfeH00tvJk98uJ"; //Fake, replace with your own Stripe secret key $stripe = new \Stripe\StripeClient($secret_key); //Add a order token for extra security $security_token = wp_generate_uuid4(); $domain = home_url(); //Create a Stripe session $checkout_session = $stripe->checkout->sessions->create([ 'payment_method_types' => ['card'], 'line_items' => [[ 'price_data' => [ 'currency' => 'cad', 'product_data' => [ 'name' => 'T-Shirts', ], 'unit_amount' => $final_price, ], 'quantity' => 1, //Stripe will mulitply the final price by the quantity so leave this set to 1 if required otherwise enter your desired quantity ]], 'mode' => 'payment', 'success_url' => $domain . '/payment-success/?order_id=' . $order_id . '&token=' . $security_token, 'cancel_url' => $domain . '/payment-cancelled?order_id=' . $order_id . '&token=' . $security_token, ]); //Optional - this can be used to send a response back to Javascript echo json_encode(array( 'status' => 'success', 'checkout_url' => $checkout_session->url )); } catch(Exception $e) { //Handle errors here //Optional - this can be used to send a response back to Javascript echo json_encode(array( 'status' => 'error', 'error' => $e->getMessage() )); }
And that’s pretty much all there is to it.
If you have any questions or require help with your web project feel free to get in touch with me at leo@digitalodyssey.ca
Cheers.