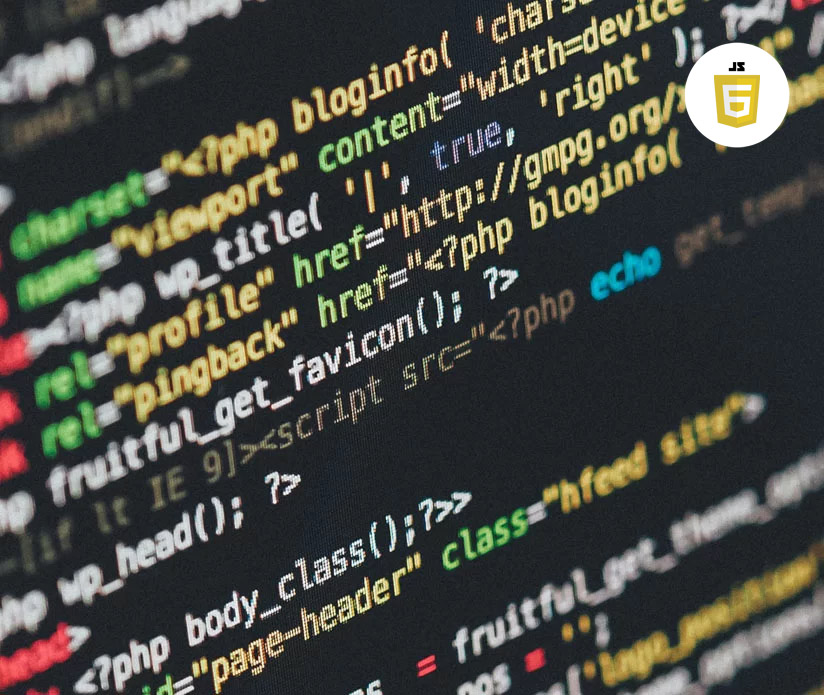
Adding Woocommerce Support to your WordPress Theme
Adding official Woocommerce support to your custom WordPress is pretty simple – you just need to add the following line of code to ensure your theme outputs Woocommerce correctly.
add_theme_support(‘woocommerce’);
Failing to declare this directive, somewhere in your functions.php file, may result in unexpected issues such as the reviews tab not appearing on the product template page. The Woocommerce plugin also offers many actions and filters that you can integrate into your WordPress theme if you need to make customizations.
Below you can find all the code with explanations that i recently covered in a Youtube video.
if (is_plugin_active('woocommerce/woocommerce.php')) { //Official declaration for woocommerce support (this is required or else you may run into issues such as the reviews tab not rendering) add_theme_support('woocommerce'); //Captures a new order add_action('woocommerce_order_status_completed', 'ln_woo_order_completed'); //Assign a custom role to a new customer add_filter('woocommerce_new_customer_data', 'ln_assign_customer_role'); //Process order refund or cancellation add_action('woocommerce_order_status_refunded', 'ln_woo_order_refunded'); add_action('woocommerce_order_status_cancelled', 'ln_woo_order_refunded'); //Check if an order has already been purchased to prevent subsequent orders add_filter('woocommerce_add_to_cart_validation', 'ln_woo_check_previous_purchase', 10, 2); //If a product has a price of zero then display the word "FREE" add_filter('woocommerce_get_price_html', 'ln_woo_price_free_zero_empty', 9999, 2); //Hide certain terms such as the default "uncategorized" add_filter('woocommerce_product_categories', 'ln_hide_selected_terms', 10, 2); //Remove certain buttons from the woocommerce navigation add_filter('woocommerce_account_menu_items', 'ln_unset_woo_menu_items'); //Disable Woocommerce breadcrumb navigation add_action( 'init', 'pm_ln_remove_wc_breadcrumbs' ); //Disable default wrapper on Woocommerce shop page remove_action('woocommerce_before_main_content', 'woocommerce_output_content_wrapper', 10); remove_action('woocommerce_after_main_content', 'woocommerce_output_content_wrapper_end', 10); //Add custom wrapper to Woocommerce shop page add_action('woocommerce_before_main_content', 'pm_ln_woo_wrapper_start', 10); add_action('woocommerce_after_main_content', 'pm_ln_woo_wrapper_end', 10); //Disable woocommerce sidebar from shop and single product templates remove_action('woocommerce_sidebar', 'woocommerce_get_sidebar', 10); //Render custom content after Woocommerce tab system on the single product page add_action('woocommerce_product_after_tabs', 'ln_custom_content_after_woocommerce_tabs', 9999, 2); } function ln_woo_order_completed($order_id) { // Get an instance of the order object $order = wc_get_order($order_id); if ($order) { //Loop through each item purchased foreach ($order->get_items() as $item_id => $item) { //Get the product id $product_id = $item->get_product_id(); //Add your code here to process custom data related to the order item } } } function ln_assign_customer_role($args) { $args['role'] = '';//Define a custom role here return $args; } function ln_woo_order_refunded($order_id) { $order = wc_get_order($order_id); if ($order) { //Add custom code here to process a refund } } function ln_woo_check_previous_purchase($passed, $product_id) { //Add custom code here to check if the user has previously purchased an item //The $passed parameter must be passed back to Woocommerce return $passed; } function ln_woo_price_free_zero_empty( $price, $product ) { //If the price is empty or equivalent to zero then return "FREE" for the output if ( '' === $product->get_price() || 0 == $product->get_price() ) { $price = '<span class="wooc-price-amount amount amount-free">'. esc_attr("FREE", "kindel-lms") .'</span>'; } return $price; } function ln_hide_selected_terms($html, $product) { //Don't render the "uncategorized" category on products if (has_term('uncategorized', 'product_cat', $product->get_id())) { return ''; } return $html; } function ln_unset_woo_menu_items( $items ) { unset($items['downloads']);//Remove the Downloads menu item return $items; } function pm_ln_woo_wrapper_start() { echo '<div class="container">'; echo '<div class="row">'; echo '<div class="col-lg-12 col-md-8 col-sm-8">'; echo ''; } function pm_ln_woo_wrapper_end() { echo '</div>'; echo '</div>'; echo '</div>'; echo ''; } function ln_custom_content_after_woocommerce_tabs() { //Get the Post ID of the product global $post; $post_id = $post->ID; //Add your code here to render content immediately after the Woocommerce tabs } function pm_ln_remove_wc_breadcrumbs() { remove_action( 'woocommerce_before_main_content', 'woocommerce_breadcrumb', 20, 0 ); }
There are many more actions and filters available which you can learn more about from the official Woocommerce developer documentation links below:
https://woocommerce.com/document/introduction-to-hooks-actions-and-filters/
https://woocommerce.github.io/code-reference/index.html
If you have any questions or require help with your web project feel free to get in touch with me at leo@digitalodyssey.ca